# renderWikiLink
This function generates an HTML anchor (<a>) element for a given title. The title is provided as an argument. commit
renderWikiLink : String -> Html msg renderWikiLink title = {- Links are enclosed in doubled square brackets Ref: Wikilinks (internal links) https://en.wikipedia.org/wiki/Help:Link and http://ward.bay.wiki.org/view/internal-link -} let target = -- title asSlug title |> String.toLower |> String.replace " " "-" |> (\s -> "/" ++ s) in a [ Html.Attributes.href target ] [ text title ]
Example: If title is "Federated Wiki", renderWikiLink should generate an Internal Link, an anchor tag like
<a class="internal" href="/federated-wiki.html" data-page-name="federated-wiki" title="view">Federated Wiki</a>
Note: Regarding title asSlug, see source view of css-spikes.dbbs.co , line 16:
const asSlug = text => text.replace(/\s/g, '-').replace(/[^A-Za-z0-9-]/g, '').toLowerCase()
# renderStory
The Render Story function is responsible for rendering a Story item into HTML format. Here we focus on Paragraph type items.
For a Paragraph type, it processes the paragraph's text field.
It applies paragraphTextAsList to paragraph.text, which converts the text into a list of strings.
renderStory : Story -> Html msg renderStory story = case story of Paragraph paragraph -> case paragraph.type_ of "paragraph" -> let textWithLinks = paragraph.text |> paragraphTextAsList |> List.map renderWikiLink in Html.p [] textWithLinks _ -> Html.text ("⚠️ INFO Paragraph – Unknown story item type: " ++ paragraph.type_) […]
Then, it maps renderWikiLink over this list of strings. This means it applies renderWikiLink to each string in the list.
Finally, it wraps the resulting list of anchor elements in an HTML paragraph (<p>) element.
paragraphTextAsList takes an input string and wraps it in a list. This is used to convert a paragraph text into a list of strings (for rendering purposes).
paragraphTextAsList : String -> List String paragraphTextAsList input = [ input ]
Note: In particular, explain the interaction of renderStory and paragraphTextAsList with respect to my attempt to develop a parser renderer for paragraphs that displays wiki links (for example: Federated Wiki) as HTML anchors.
Example: Suppose you have a Paragraph with text equal to "This is a sample link in a paragraph". The renderStory function will:
Apply paragraphTextAsList and convert the text into ["This is a sample link in a paragraph"].
Apply renderWikiLink to each element in the list, generating HTML anchor elements for the link. This should result in something like ["This is a <a href="/sample-link.html">sample link</a> in a paragraph"].
Wrap this list in an HTML paragraph element, resulting in <p>This is a <a href="/sample-link.html">sample link</a> in a paragraph</p>.
At the moment, the whole paragraph is output as a wiki link (see figure below), not just the links enclosed in double square brackets.
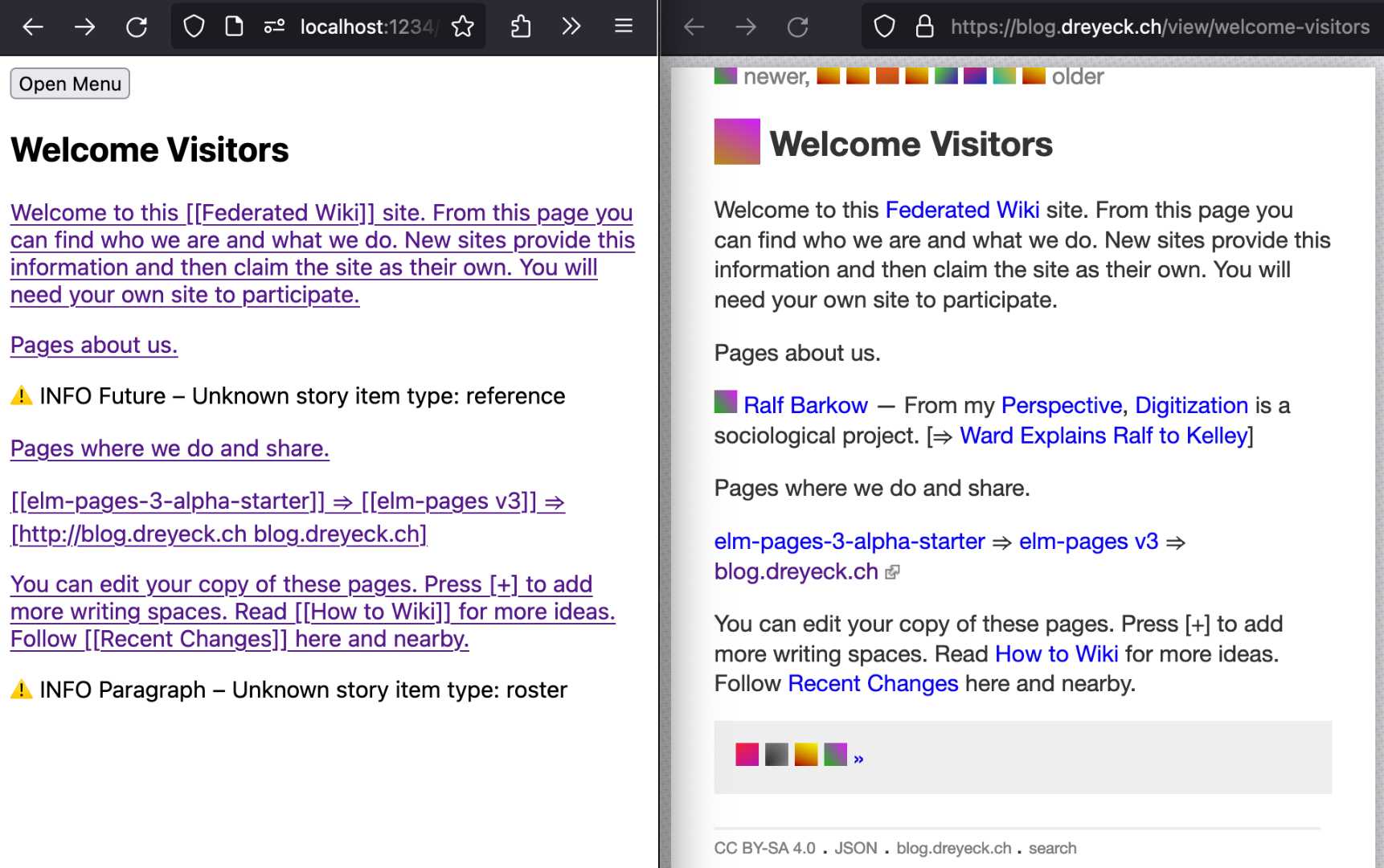
Whole paragraph asWikiLink
For example, the false-positive "Page about us." targeting http://localhost:1234/pages-about-us. – although there is no wiki link in the paragraph at all.
In summary, renderStory utilizes paragraphTextAsList to convert the text of a paragraph-like item into a list of strings. It then applies renderWikiLink to each string in the list to generate HTML anchor elements for wiki links. This allows for the rendering of paragraphs with clickable wiki links.
How would the code have to be changed to render only the links as anchors? Maybe with a parser renderer for wiki links?
To render only the links enclosed in double square brackets as anchors, you'll need to implement a parser/renderer for wiki links. This parser should be able to identify the links within the text and convert them into HTML anchor elements.
Here's how you can do it:
1. Create a Parser/Renderer for Wiki Links: You'll need a function that can identify and convert wiki links within a string. This function should take a string as input and return a modified string with the links converted to HTML anchor elements. For example, you might create a function called parseWikiLinks:
parseWikiLinks : String -> Html msg
This function would take a string and return an Html element representing the parsed content.
2. Modify renderStory: In your renderStory function, when dealing with a Paragraph item, apply the parseWikiLinks function to the paragraph's text.
renderStory : Story -> Html msg renderStory story = case story of Paragraph paragraph -> case paragraph.type_ of "paragraph" -> let renderedText = paragraph.text |> parseWikiLinks in Html.p [] [ text renderedText ] _ -> Html.text ("⚠️ INFO Paragraph – Unknown story item type: " ++ paragraph.type_) -- Handle other cases as needed...
The parseWikiLinks function will process the text and return a modified string with wiki links converted to HTML anchors. You'll need to define this function separately.
3. Define parseWikiLinks: In this function, you'll need to implement the logic for identifying and converting wiki links into HTML anchor elements. This could involve using regular expressions, string manipulation, or any other parsing technique you find suitable.
parseWikiLinks : String -> Html msg parseWikiLinks input = -- Implement the logic to identify and convert wiki links -- Return an HTML element representing the parsed content
Inside this function, you'll need to iterate over the input string, identify [⇒ Gobble It Up] links enclosed in double square brackets ([[...]]), and replace them with corresponding HTML anchor elements.
Remember, the specific implementation of parseWikiLinks will depend on your exact requirements and how your wiki links are formatted in the input text. You may need to use regular expressions or other parsing techniques to achieve the desired result. (ChatGPT August 3 Version)
⇒ MiniLatex: a Parser-Renderer for a Subset of LaTeX pdf ⇐ Do Internal Link
~
wikiLinkParser : Parser WikiLink wikiLinkParser = succeed WikiLink |. symbol "[[" |= (getChompedString <| chompWhile (\c -> c /= ']')) |. symbol "]]"
getChompedString search