We explore solutions to a Martin Gardner puzzel using math and then modeling it with CAD software with no real intention to 3d print our solutions.
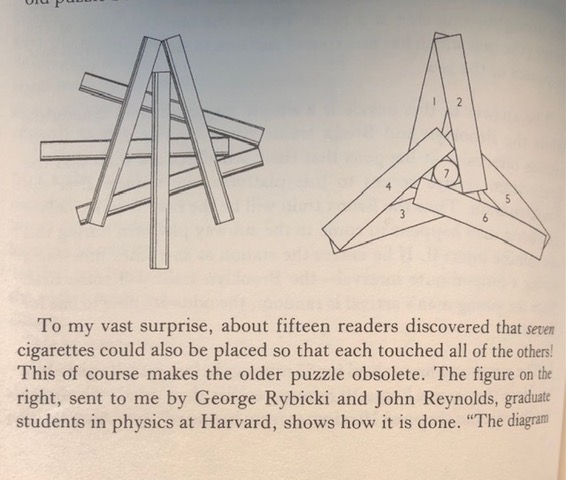
From My Best Mathematical and Logic Puzzles. dover
analysis
The problem: Six cylinders (pencils or cigarettes) arranged so that each one touches all five of the others.
I will describe this in the same manner as you were doing with your cad program.
Start with a cylinder of radius R, length L. The center of the base of the cylinder is at the origin. I’ll take the horizontal axis as x and the vertical as y, with z coming out of the screen toward the viewer.
Assume: L increases in the +y direction.
module pencil() { rotate([-90,0,0]) cylinder(h=L,r=R); }
# 1. Triangle 1
Make a copy of the cylinder.
Rotate that cylinder to the right by angle w.
Translate the cylinder: In the x direction by R * cos w. In the y direction by - R * (sin w + 1/tan w).
translate([R*cos(w), -R*(sin(w)+1/tan(w)), 0]) rotate([0,0,-w]) pencil();
This translates it down and to the right.
Copy the original cylinder. Rotate this one by an angle -w. Translate it as before but reverse the x direction to -R * cos w. This translates it down and to the left.
translate([-R*cos(w), -R*(sin(w)+1/tan(w)), 0]) rotate([0,0,-(-w)]) pencil();
The three cylinders form one “triangle” of the sort you wrote before. Each cylinder touches the other two.
For the specific “equilateral” case where the rotation is by w = 30 degrees, the angled cylinders have the origin of their base at X = +/- 0.866 * R Y = 0.5 * R (Using sin w = 1/2, cos w = sqrt(3)/2.)
# 2. Triangle 2.
Make a copy of Triangle 1. Shift it in the z direction by 2*R so the new triangle sits atop the first triangle.
Now one has to make choices about how one wants the result to look. The most symmetric choice is the following:
Rotate the second triangle by 90 degrees clockwise about the origin. So the base of the middle cylinder is still centered on the origin.
Translate the second triangle up and to the left by an amount D each way. So Translate in x direction by -D. Translate in y direction by D.
The choice of D determines whether all the cylinders touch or not, and how much of an overlap there is. Let’s denote by f the point where the two most separated cylinders touch. This is expressed as a fraction of the cylinder length. So to touch, we need 0 < f < 1.
If f = 1, the two farthest cylinders just touch at their farther tips. If f > 1, the cylinders won’t touch.
This fraction f depends on the cylinder dimensions R and L, and the choice of translation point D (used above).
The formula for f is
f = a * D/L + b * R/L
Where a and b depend on the rotation angle w. For the case of w = 30 degrees,
a = 2.7322
b = 4.7324
In general,
a = 1/(cos w - sin w)
b = a/(tan w)
By choosing a pleasing value for f, for example, f = 0.78, one finds the relation between cylinder length, radius and crossing point (D) that enables all six cylinders to touch each of the other.
Choosing f, R, L, and D also places a restriction on the point of touch of the two cylinders closest to each other.
a = 1/(cos(w) - sin(w)); b = a/(tan(w)); f = 0.8; D = (f - b * R/L)*L/a; triangle(); translate([-D,D,2*R]) rotate([0,0,-90]) triangle();
For the 30 degree case, this touching point is at 0.27 * f of the length of a cylinder. (Choosing f=0.78 makes this nearest touching point have the same overlap as the farthest touching point in this case.)
implementation
We're very close but can't find a way to close the final gap without opening another gap.
pages/touching-cylinders
See OpenSCAD
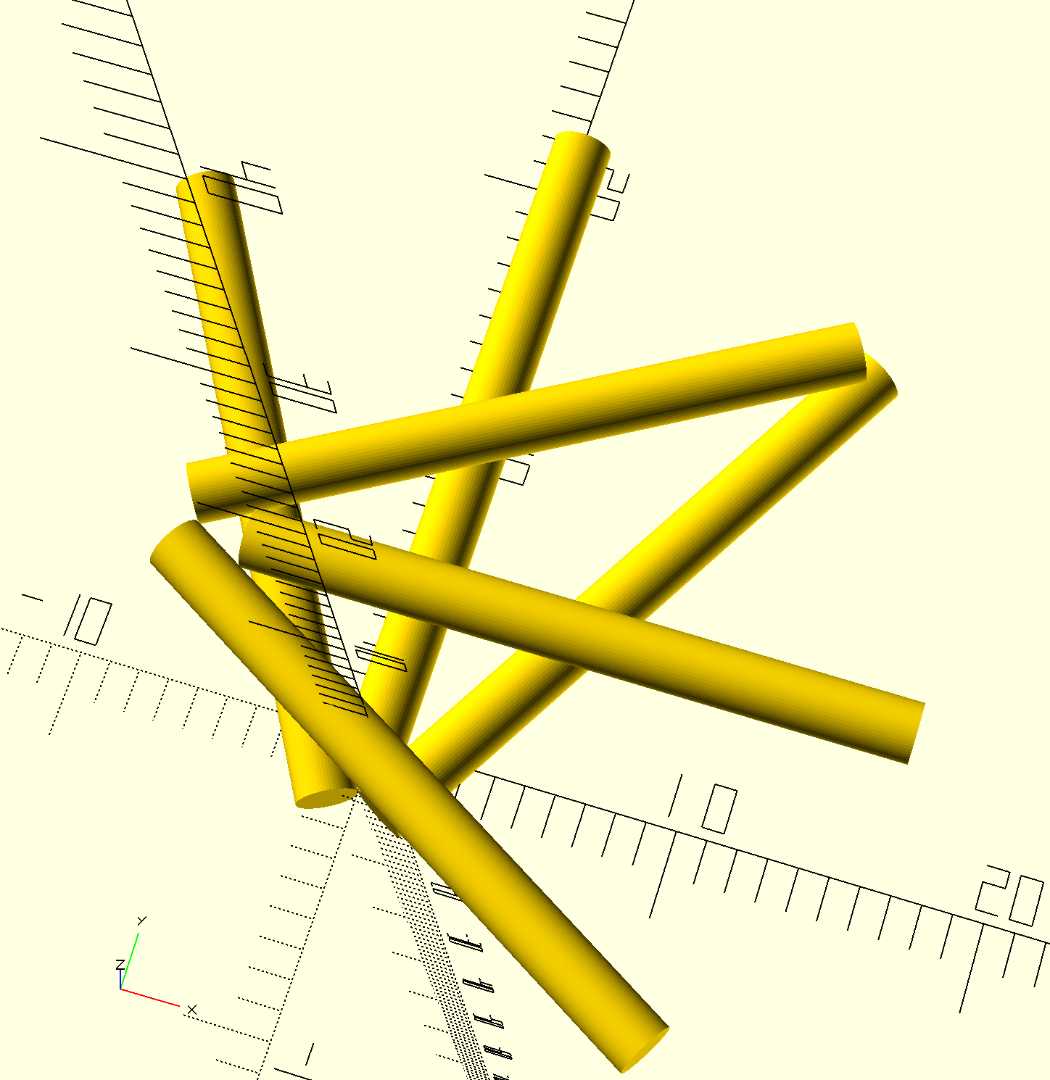
Uploaded image
Derivation
Consider a cylinder of radius R, length L, with the center of its base at the origin, and oriented along the +y axis.
Copy the cylinder and shift the copy in the +z direction by 2R. Then this cylinder will touch the initial cylinder along a line in the y direction. Now rotate the copy by an angle u with respect to the +y direction and translate the center of its base by (-C, D), where for definiteness we set C >= 0, D >= 0, and 0<=u<=180 degrees. The original line of contact on the copy now has the equation
y = m(x + C) + D
where m = 1/tan u. Because the cylinder has length L and is at angle u to the y axis, x is bounded by
-C < x < -C + L * sin u
The line of contact on the original cylinder had equation (0,y) for 0 < y < L. The rotated line on the copy can only intersect the line on the original cylinder (both at z = R) where x = 0, so y = mC + D. This provides a constraint among the shift variables and L,
0 < mC + D < L.
that needs to be met in order for there to be a point of contact. This provides an example of how constraints are found for the touching points. Now we construct the assemblage and find all the needed constraints.
In the z=0 plane we can make a "triangle" of three cylinders that mutually touch, as described in the implementation section. Choose an angle w > 0 and define the positive quantities
a = R * cos w
b = R * (sin w + 1/tan w)
Rotate a copy of the original cylinder by w and translate the center of its base to (a, -b). Rotate a second copy of the original cylinder by -w and translate it by (-a, -b). The three cylinders so made will each touch each other in the z=0 plane. The line of contact with the z=R plane has the equation
y = n(x-a) - b
for the clockwise rotated cylinder of Triangle 1, with the constraint
a < x < a + L * sin w
And for the other, the counterclockwise rotated cylinder of Triangle 1,
y = -n(x+a) - b,
- a - L * sinw < x < - a
In both cases, n = 1/tan w. These copies and the original cylinder make up Triangle 1. They mutually touch.
We similarly make three touching cylinders in the z = R plane, by rotating by angle u and translated by (-C, D). These have the following equations and constraints:
Central cylinder, Triangle 2 (as above):
y = m*(x+C) + D, -C < x < -C + L * sin u, m = 1/tan u
Clockwise rotated cylinder, Triangle 2:
y = m'*(x+C+b) + (D-a), -C-b < x < -C-b+L*sin(u+w), m' = 1/tan(u+w)
Counterclockwise rotated cylinder, Triangle 2:
y = m''*(x+C+b) + (D+a), -C-b < x < -C-b+L*sin(u-w), m'' = 1/tan(u-w)
To mutually touch, the equations for each of these line segments in the z = R plane must share a point with each of those in the z = 0 plane. That makes 9 constraints. However, using symmetry, we can see that we can reduce the number of constraints. First, because the vertex of Triangle 2 was chosen to be in the upper left quadrant, if the two non-central cylinders of Triangle 2 each are ensured to touch the furthermost (clockwise rotated) cylinder of Triangle 1, then the central cylinder must also. Similarly, if the central cylinder of Triangle 2 is ensured to touch the nearest (counterclockwise rotated) cylinder of Triangle 1, the cylinders will also touch it. touch. This uses the fact that the vertex of Triangle 2 is in the upper left quadrant while that of Triangle 1 is along the negative y axis.
This gives three constraint equations by equating the y values for two of the lines, solving for x, and applying the constraints on x to arrive at constraints on the six values R,L,u,w,C and D.
The three x values for these touch points are:
x1 = -(mC+D+na+b)/(m+n)
x2 = -(m''(C+b)+D+na+b+a)/(m''-n)
x3 = -(m'(C+b)+D+na+b-a)/(m'-n)
These are, respectively, the touch points Central 2
touching CCW1, CCW2 touching CW1, and CW2 touching CW1. These have constraints (after some common sense simplifications),
x1 < -a
x1 > max(-C, -a - L*sin w)
x2 < min(-C-b+L*sin(u+w), a+L*sin w)
x2 > a
x3 < min(-C-b+L*sin(u-w), a+L*sin w)
x3 > a
Substituting in the values of the x points gives constraint equations on combinations of the quantities R,L,u,w,C and D.
Particular Solutions (unfinished)
Because there are six variables and three combinations that are constrained, we should be free to select the values of three variables. For a physical problem we would usually be given R and L, for example, R/L = 1/20. We can search for a symmetric solution with the two triangles at right angles to each other. Through trial in Excel, one solution that satisfies the constraints is:
R = 1, L = 20, u = 90 deg
w = 15 deg, C = 5, D = 5
The touch points are (approximately)
x1 = -3.6, y1 = 5
x2 = 4.9, y2 = 9.87
x3 = 2.4, y3 = 0.78
------