recommend | BrE ˌrɛkəˈmɛnd, AmE ˌrɛkəˈmɛnd | transitive verb 1 empfehlen ▸ recommend sb to do sth jmdm. empfehlen, etw. zu tun 2 (make acceptable) sprechen für ▸ the plan has little/nothing to recommend it es spricht wenig/nichts für den Plan
~
The `recommend` function of the Solo Super Collaborator is used to recommend similar items based on a chosen set of items represented by a graph.
function recommend(chosen) { const vocabulary = new Set() const hash = node => `${node.type}: ${node.props.name || ''}` for (const poem of chosen) { for (const node of poem.graph.nodes) { vocabulary.add(hash(node)) } } const similar = graph => { for (const node of graph.nodes) { if (vocabulary.has(hash(node))) return true } return false } const beam = croquet.view.beam() window.beam.querySelectorAll('input[type=checkbox]').forEach(elem => { const color = !elem.checked && similar(beam[elem.value].graph) ? 'darkorange' : 'black' elem.nextElementSibling.style.color = color }) }
Here's how it works: 1. It initializes an empty set called `vocabulary` to Store Unique Representations of Nodes. 2. It defines a hashing function called `hash` to Generate a Unique Representation of Each Node based on its type and name (if available). 3. It iterates over each Poem in the `chosen` array and for each node in each poem's graph, it adds the hashed representation of the node to the `vocabulary` set. 4. It defines a function called `similar` which takes a graph as input and checks if any node in the graph has a hashed representation that matches any in the `vocabulary` set. If such a match is found, it returns `true`; otherwise, it returns `false`. 5. It retrieves the `beam` from a `croquet.view` object (assuming `croquet.view.beam()` returns the beam) and iterates over each checkbox input element within the `beam`. 6. For each checkbox, it determines whether it is similar to the chosen items by calling the `similar` function with the corresponding graph from the `beam`. 7. If the checkbox is not checked and it is similar to any of the chosen items, it sets the color of its next sibling element (presumably a label or text) to 'darkorange'; otherwise, it sets it to 'black'. In summary, this function recommends similar items in a user interface by visually highlighting them based on their Similarity to the chosen items. (ChatGPT 3.5)
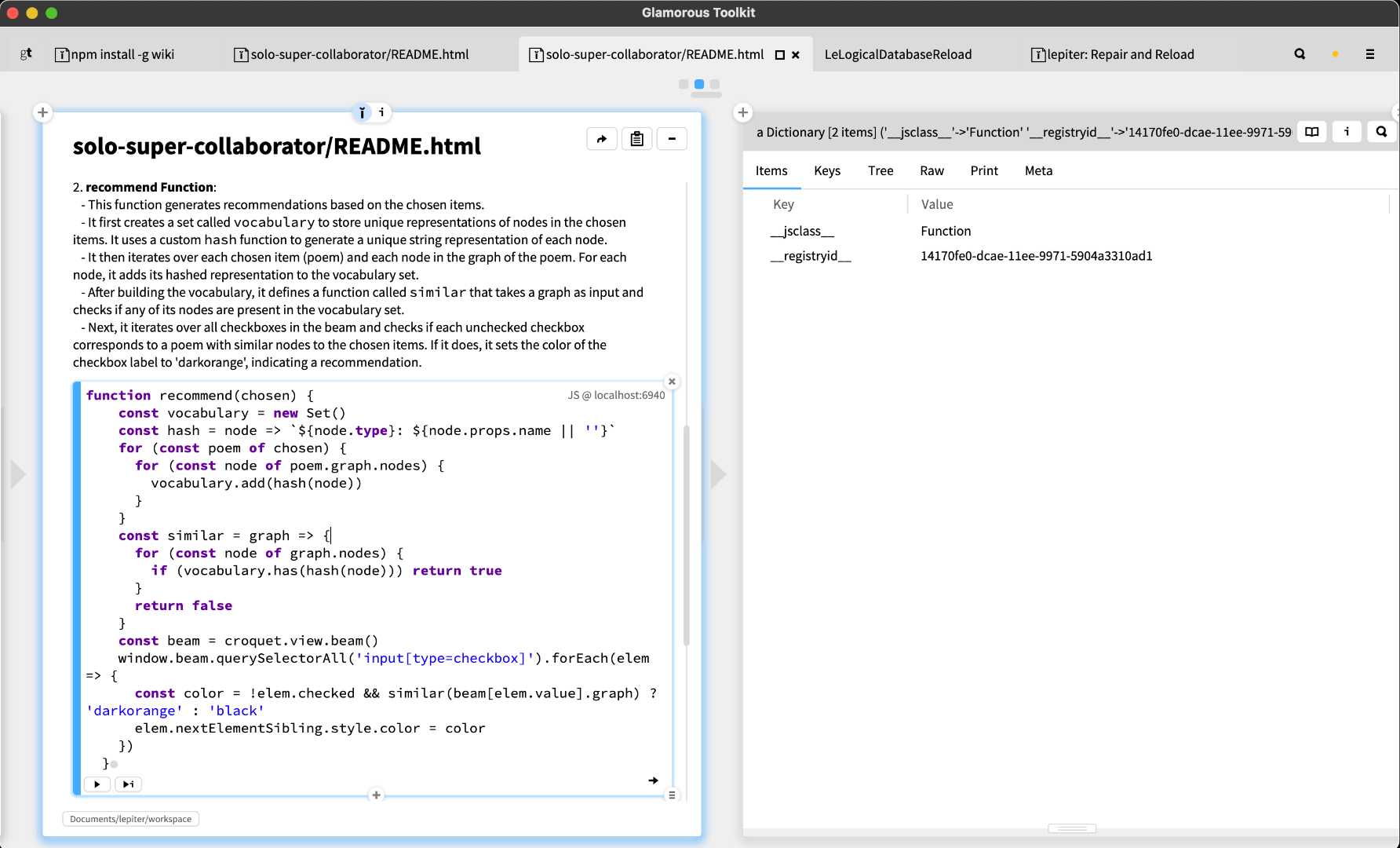
function recommend
# Assets
pages/recommend
⇒ Solo Super Collaborator with recommend's new similar function. Try it. readme
const similar = (graph) => { for (const node of graph.nodes) { if (vocabulary.has(hash(node))) { node.props["recommended"] = true; return true; } } // If no similar node found, set "recommended" property to false for (const node of graph.nodes) { node.props["recommended"] = false; } return false; }
⇒ Property Graphs as Javascript Module: How to add a key-value pair to the property map of a node? commit